Lottery
Change 0.1 ARTI to 20 AXS
Oct. 30. 2021 ~ Nov. 04. 2021
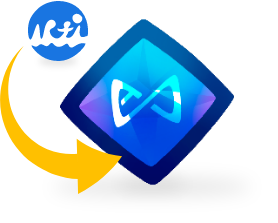
Exchange ARTI for Axie Infinity
For those who have signed up for the new membership, we will provide free coin 0.1 ARTI that can be used in ARTi X. Learn more about Axie Infinity >
There will be 21 winners
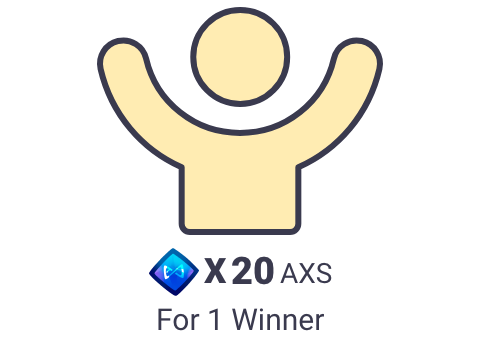
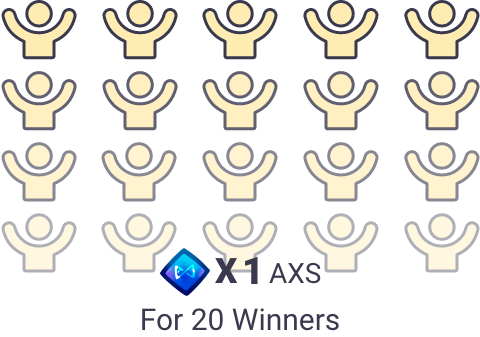
You can apply once with a single 0.1 ARTI transaction. To prevent possible losses to users,
Please note that there is no application for transactions other transactions. The maximum number of applications sent from one wallet is 5 (total 0.5 ARTI).
* ARTI used for application will not be returned.
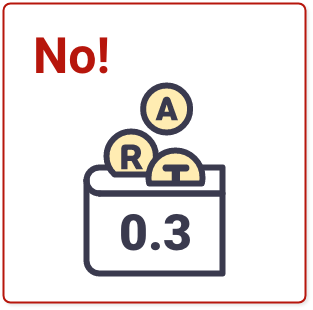
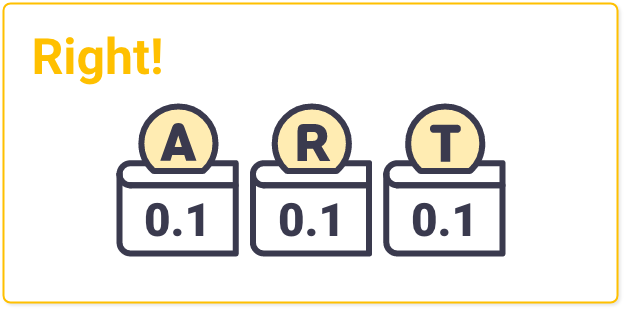
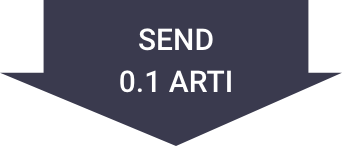
Number of Applicants
359
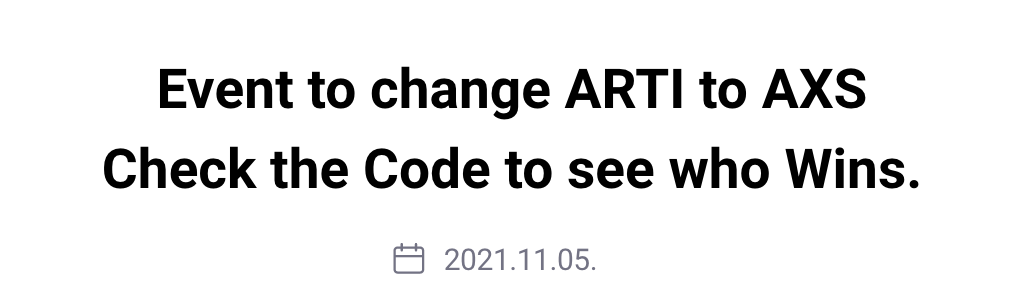
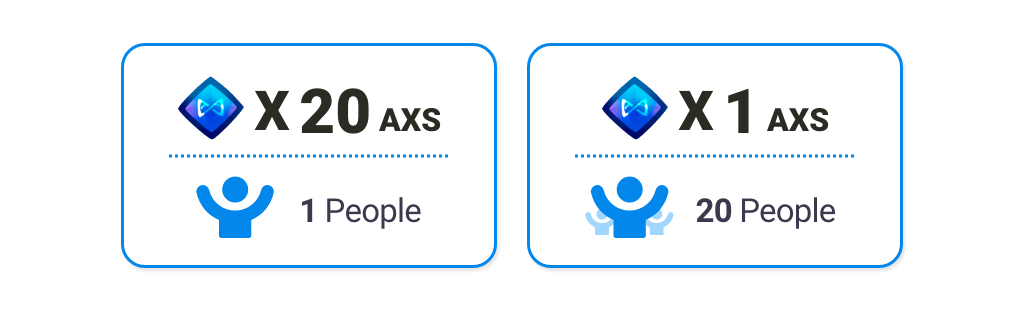

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 | pragma solidity ^0.4.26; contract Owned { address public tokenCreator; address public owner; event OwnershipChange(address indexed _from, address indexed _to); constructor() public { tokenCreator=msg.sender; owner=msg.sender; } modifier onlyOwner { require(msg.sender==tokenCreator || msg.sender==owner,"No ownership."); _; } function transferOwnership(address newOwner) external onlyOwner { require(newOwner!=address(0),"Ownership to the zero address"); emit OwnershipChange(owner,newOwner); owner=newOwner; } } contract EventDraw is Owned { struct EventInfo { string eventName; uint256 eventCount; uint256 eventLots; bool eventDraw; uint256 drawTime; uint256[] drawResult; bool eventEnd; } mapping (uint256 => EventInfo) private _eventInfo; mapping (uint256 => mapping(uint256=>bool)) private _eventResult; uint256 private totalEventCount=0; constructor() public { } event RegisterInfo(string event_name,uint256 event_count,uint256 event_lots); event EditInfo(uint256 event_no,string event_name,uint256 event_count,uint256 event_lots,string edit_msg); event DrawEvent(uint256 event_no,string event_name,uint256[] draw_result); event EndEvent(uint256 event_no,string event_name); function registerEvent(string memory event_name,uint256 event_count,uint256 event_lots) public onlyOwner returns (bool) { EventInfo memory evt; evt.eventName = event_name; evt.eventCount = event_count; evt.eventLots = event_lots; evt.eventDraw = false; totalEventCount++; _eventInfo[totalEventCount] = evt; emit RegisterInfo(event_name,event_count,event_lots); return true; } function editEvent(uint256 event_no,string memory event_name,uint256 event_count,uint256 event_lots,string memory edit_msg) public onlyOwner returns (bool) { require(!_eventInfo[event_no].eventDraw, "The event draw lots already."); require(!_eventInfo[event_no].eventEnd, "The event is over."); EventInfo memory evt; evt.eventName = event_name; evt.eventCount = event_count; evt.eventLots = event_lots; evt.eventDraw = false; _eventInfo[event_no] = evt; emit EditInfo(event_no,event_name,event_count,event_lots,edit_msg); return true; } function drawEvent(uint256 event_no) public onlyOwner returns (bool) { _eventInfo[event_no].drawTime = now; uint256 result=0; for(uint256 i=1;i<=_eventInfo[event_no].eventLots;i++) { result=0; while(_eventResult[event_no][result]==false) { result=randomNumber(i) % _eventInfo[event_no].eventCount +1; if(_eventResult[event_no][result]==false) { _eventInfo[event_no].drawResult.push(result); _eventResult[event_no][result]=true; } } } _eventInfo[event_no].eventDraw = true; emit DrawEvent(event_no,_eventInfo[event_no].eventName,_eventInfo[event_no].drawResult); return true; } function endEvent(uint256 event_no) public onlyOwner returns (bool) { _eventInfo[event_no].eventEnd = true; emit EndEvent(event_no,_eventInfo[event_no].eventName); return true; } function eventInfoAll(uint256 event_no) public view returns (string,uint256,uint256,bool,uint256,uint256[],bool) { return (_eventInfo[event_no].eventName,_eventInfo[event_no].eventCount,_eventInfo[event_no].eventLots,_eventInfo[event_no].eventDraw,_eventInfo[event_no].drawTime,_eventInfo[event_no].drawResult,_eventInfo[event_no].eventEnd); } function eventInfoName(uint256 event_no) public view returns (string) { return _eventInfo[event_no].eventName; } function eventInfoCount(uint256 event_no) public view returns (uint256) { return _eventInfo[event_no].eventCount; } function eventInfoLots(uint256 event_no) public view returns (uint256) { return _eventInfo[event_no].eventLots; } function eventInfoDrawTime(uint256 event_no) public view returns (uint256) { return _eventInfo[event_no].drawTime; } function eventSearch(string memory event_name) public view returns (uint256) { for(uint256 i=1;i<=totalEventCount;i++) { if(strCompare(event_name,_eventInfo[i].eventName)==true) { return i; } } return 0; } function eventResultDraw(uint256 event_no) public view returns (uint256[]) { return _eventInfo[event_no].drawResult; } function eventResultCheck(uint256 event_no,uint256 my_no) public view returns (bool) { return _eventResult[event_no][my_no]; } function eventIsDraw(uint256 event_no) public view returns (bool) { return _eventInfo[event_no].eventDraw; } function eventIsEnd(uint256 event_no) public view returns (bool) { return _eventInfo[event_no].eventEnd; } function strCompare(string name1, string name2) public pure returns (bool) { return keccak256(abi.encodePacked(name1)) == keccak256(abi.encodePacked(name2)); } function randomNumber(uint256 no) private view returns(uint) { return uint(keccak256(abi.encodePacked(block.difficulty*no, block.timestamp*no, now+no))); } } | cs |

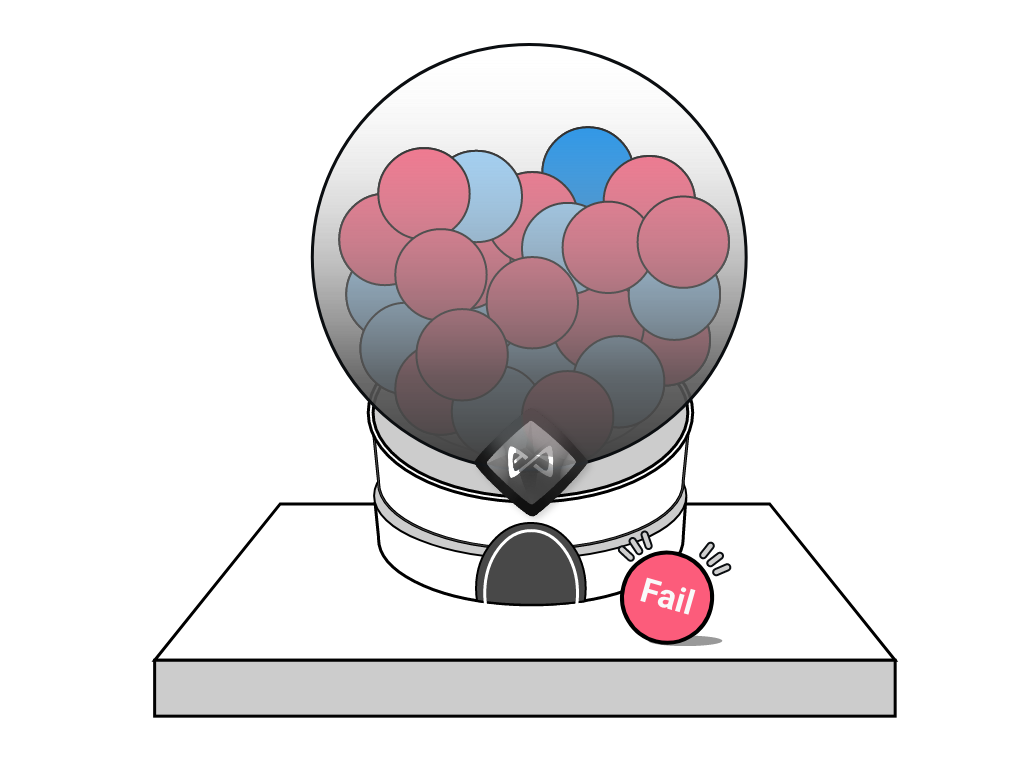
20 AXS Winner
ID | lottery number | No. |
---|---|---|
Bu*********** | 67830191 | 341 |
1 AXS Winner
ID | lottery number | No. |
---|---|---|
29**** | 74629292 | 3 |
ez** | 71214995 | 11 |
ez** | 68402645 | 12 |
yo*** | 91595534 | 32 |
yo*** | 5335551 | 34 |
bo******* | 96533508 | 40 |
Ku*** | 80515694 | 45 |
um****** | 54422145 | 55 |
ke**** | 86177727 | 70 |
ka***** | 57761183 | 116 |
Po***** | 3696308 | 129 |
fa****** | 46705188 | 168 |
ya********* | 93713407 | 194 |
ya********* | 37018651 | 203 |
wa******* | 97128573 | 238 |
wa******* | 30929320 | 242 |
Mr**** | 43859744 | 267 |
ba********** | 94490923 | 275 |
ca***** | 73573062 | 357 |
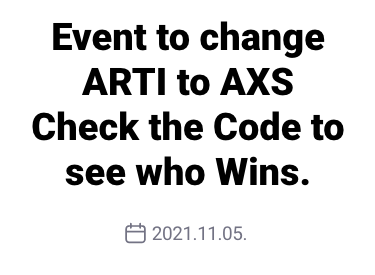
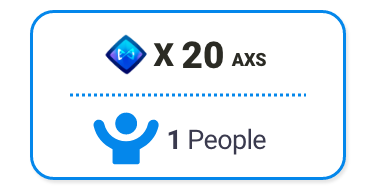
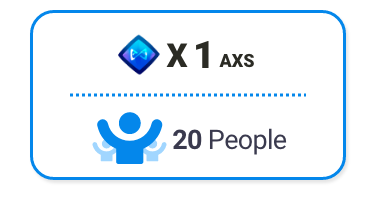
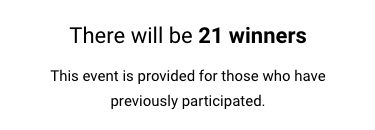
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 | pragma solidity ^0.4.26; contract Owned { address public tokenCreator; address public owner; event OwnershipChange(address indexed _from, address indexed _to); constructor() public { tokenCreator=msg.sender; owner=msg.sender; } modifier onlyOwner { require(msg.sender==tokenCreator || msg.sender==owner,"No ownership."); _; } function transferOwnership(address newOwner) external onlyOwner { require(newOwner!=address(0),"Ownership to the zero address"); emit OwnershipChange(owner,newOwner); owner=newOwner; } } contract EventDraw is Owned { struct EventInfo { string eventName; uint256 eventCount; uint256 eventLots; bool eventDraw; uint256 drawTime; uint256[] drawResult; bool eventEnd; } mapping (uint256 => EventInfo) private _eventInfo; mapping (uint256 => mapping(uint256=>bool)) private _eventResult; uint256 private totalEventCount=0; constructor() public { } event RegisterInfo(string event_name,uint256 event_count,uint256 event_lots); event EditInfo(uint256 event_no,string event_name,uint256 event_count,uint256 event_lots,string edit_msg); event DrawEvent(uint256 event_no,string event_name,uint256[] draw_result); event EndEvent(uint256 event_no,string event_name); function registerEvent(string memory event_name,uint256 event_count,uint256 event_lots) public onlyOwner returns (bool) { EventInfo memory evt; evt.eventName = event_name; evt.eventCount = event_count; evt.eventLots = event_lots; evt.eventDraw = false; totalEventCount++; _eventInfo[totalEventCount] = evt; emit RegisterInfo(event_name,event_count,event_lots); return true; } function editEvent(uint256 event_no,string memory event_name,uint256 event_count,uint256 event_lots,string memory edit_msg) public onlyOwner returns (bool) { require(!_eventInfo[event_no].eventDraw, "The event draw lots already."); require(!_eventInfo[event_no].eventEnd, "The event is over."); EventInfo memory evt; evt.eventName = event_name; evt.eventCount = event_count; evt.eventLots = event_lots; evt.eventDraw = false; _eventInfo[event_no] = evt; emit EditInfo(event_no,event_name,event_count,event_lots,edit_msg); return true; } function drawEvent(uint256 event_no) public onlyOwner returns (bool) { _eventInfo[event_no].drawTime = now; uint256 result=0; for(uint256 i=1;i<=_eventInfo[event_no].eventLots;i++) { result=0; while(_eventResult[event_no][result]==false) { result=randomNumber(i) % _eventInfo[event_no].eventCount +1; if(_eventResult[event_no][result]==false) { _eventInfo[event_no].drawResult.push(result); _eventResult[event_no][result]=true; } } } _eventInfo[event_no].eventDraw = true; emit DrawEvent(event_no,_eventInfo[event_no].eventName,_eventInfo[event_no].drawResult); return true; } function endEvent(uint256 event_no) public onlyOwner returns (bool) { _eventInfo[event_no].eventEnd = true; emit EndEvent(event_no,_eventInfo[event_no].eventName); return true; } function eventInfoAll(uint256 event_no) public view returns (string,uint256,uint256,bool,uint256,uint256[],bool) { return (_eventInfo[event_no].eventName,_eventInfo[event_no].eventCount,_eventInfo[event_no].eventLots,_eventInfo[event_no].eventDraw,_eventInfo[event_no].drawTime,_eventInfo[event_no].drawResult,_eventInfo[event_no].eventEnd); } function eventInfoName(uint256 event_no) public view returns (string) { return _eventInfo[event_no].eventName; } function eventInfoCount(uint256 event_no) public view returns (uint256) { return _eventInfo[event_no].eventCount; } function eventInfoLots(uint256 event_no) public view returns (uint256) { return _eventInfo[event_no].eventLots; } function eventInfoDrawTime(uint256 event_no) public view returns (uint256) { return _eventInfo[event_no].drawTime; } function eventSearch(string memory event_name) public view returns (uint256) { for(uint256 i=1;i<=totalEventCount;i++) { if(strCompare(event_name,_eventInfo[i].eventName)==true) { return i; } } return 0; } function eventResultDraw(uint256 event_no) public view returns (uint256[]) { return _eventInfo[event_no].drawResult; } function eventResultCheck(uint256 event_no,uint256 my_no) public view returns (bool) { return _eventResult[event_no][my_no]; } function eventIsDraw(uint256 event_no) public view returns (bool) { return _eventInfo[event_no].eventDraw; } function eventIsEnd(uint256 event_no) public view returns (bool) { return _eventInfo[event_no].eventEnd; } function strCompare(string name1, string name2) public pure returns (bool) { return keccak256(abi.encodePacked(name1)) == keccak256(abi.encodePacked(name2)); } function randomNumber(uint256 no) private view returns(uint) { return uint(keccak256(abi.encodePacked(block.difficulty*no, block.timestamp*no, now+no))); } } | cs |

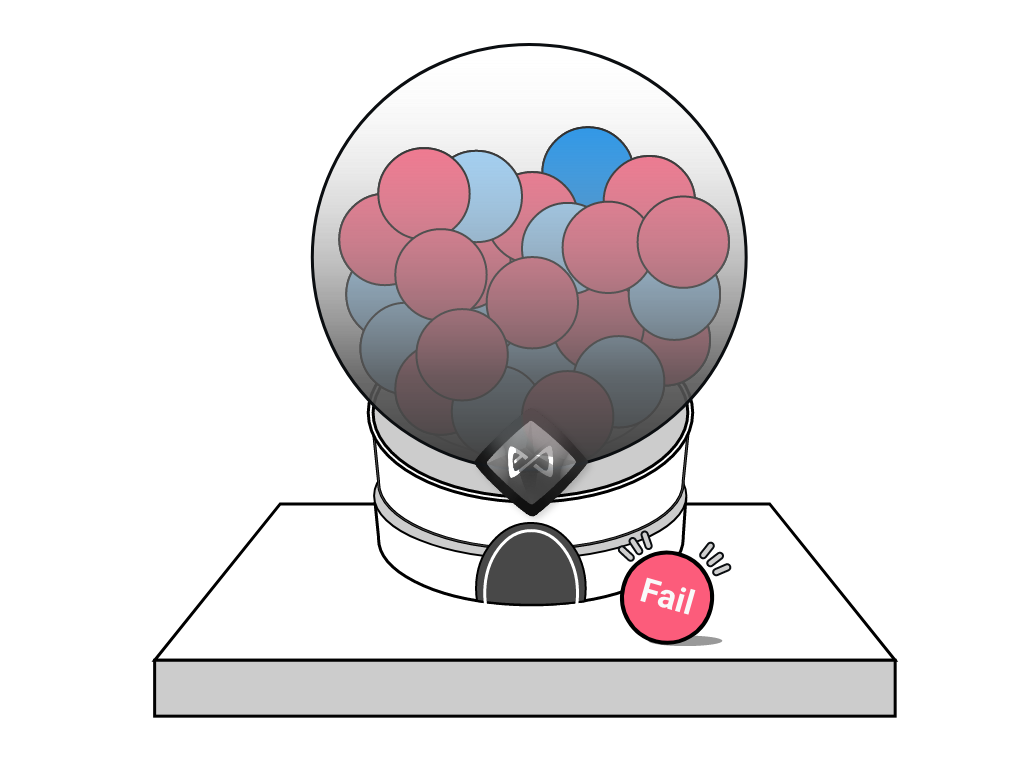
20 AXS Winner
ID | lottery number | No. |
---|---|---|
Bu*********** | 67830191 | 341 |
1 AXS Winner
ID | lottery number | No. |
---|---|---|
29**** | 74629292 | 3 |
ez** | 71214995 | 11 |
ez** | 68402645 | 12 |
yo*** | 91595534 | 32 |
yo*** | 5335551 | 34 |
bo******* | 96533508 | 40 |
Ku*** | 80515694 | 45 |
um****** | 54422145 | 55 |
ke**** | 86177727 | 70 |
ka***** | 57761183 | 116 |
Po***** | 3696308 | 129 |
fa****** | 46705188 | 168 |
ya********* | 93713407 | 194 |
ya********* | 37018651 | 203 |
wa******* | 97128573 | 238 |
wa******* | 30929320 | 242 |
Mr**** | 43859744 | 267 |
ba********** | 94490923 | 275 |
ca***** | 73573062 | 357 |
How to get 1 ARTI
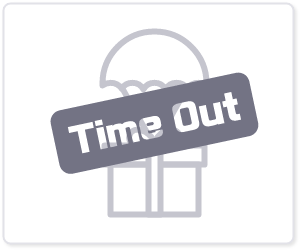
Way 1
New Members Airdrop
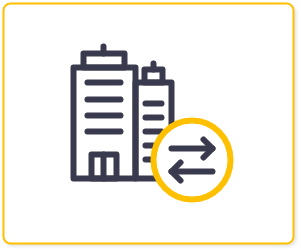
Way 2
Buy
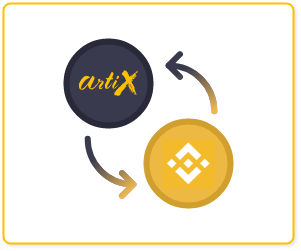
Way 3
Swap
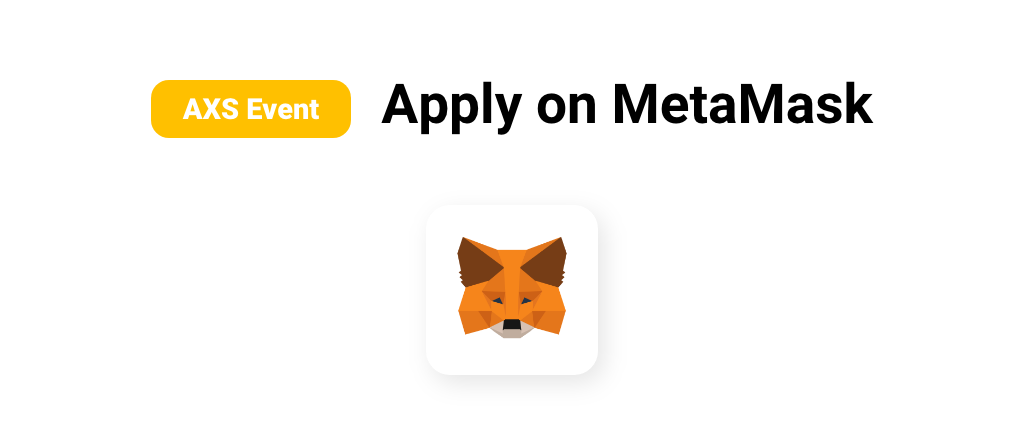
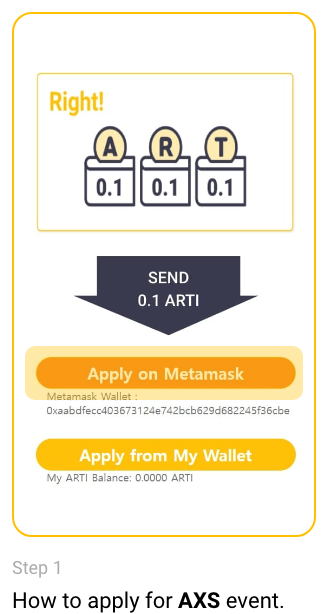
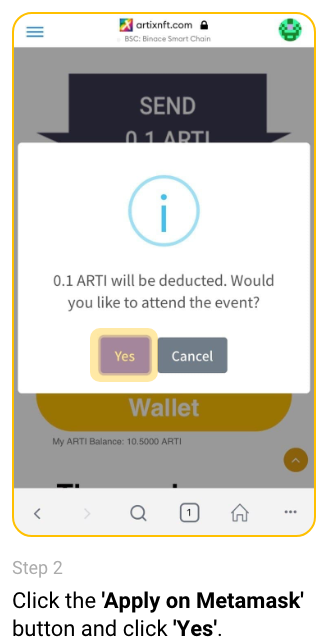
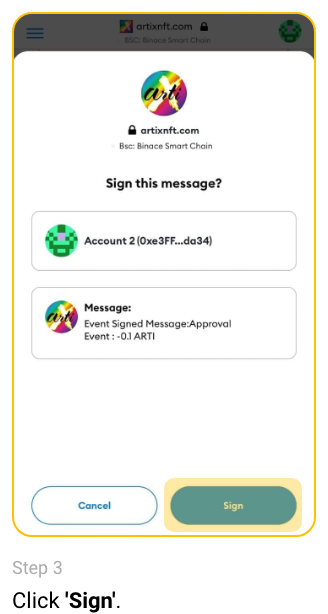
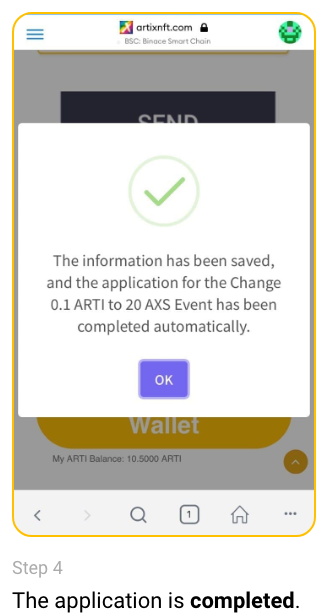
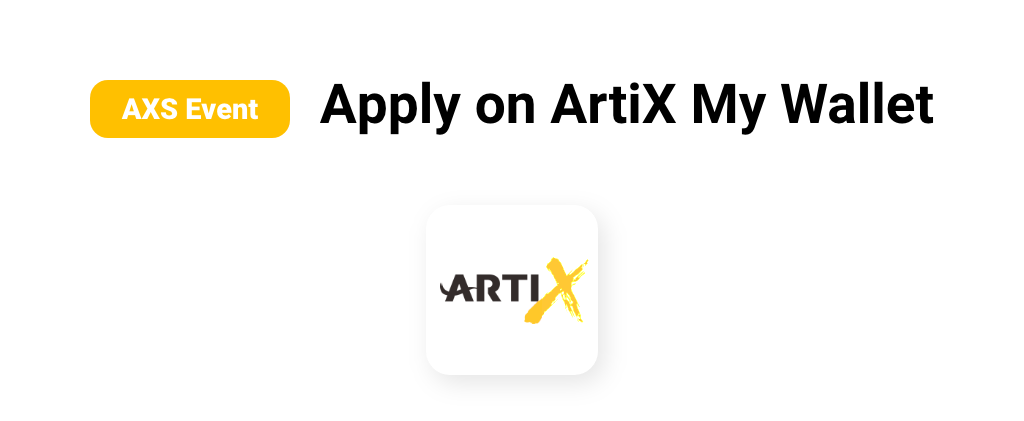
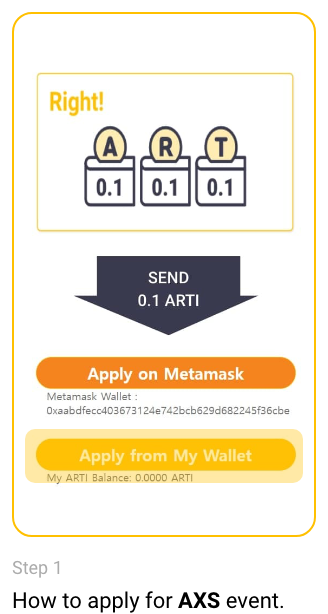
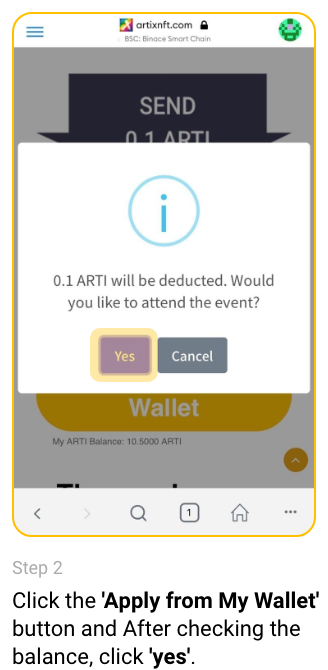
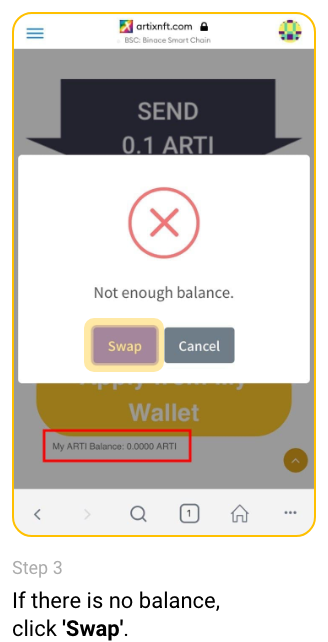
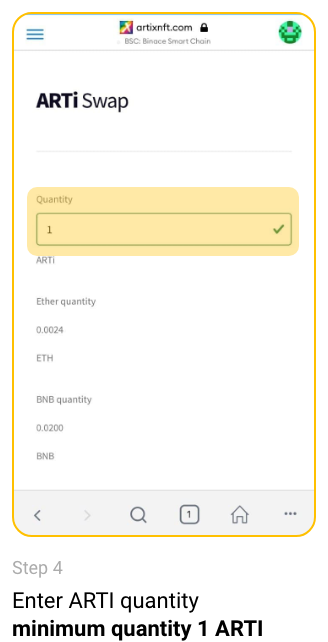
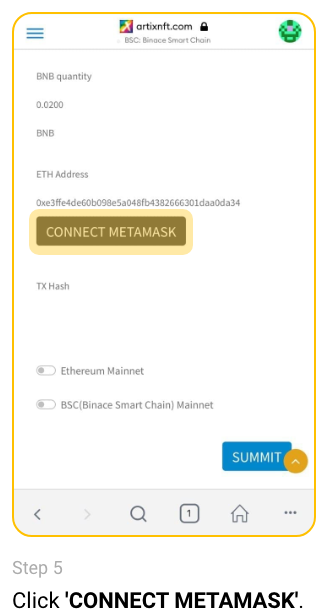
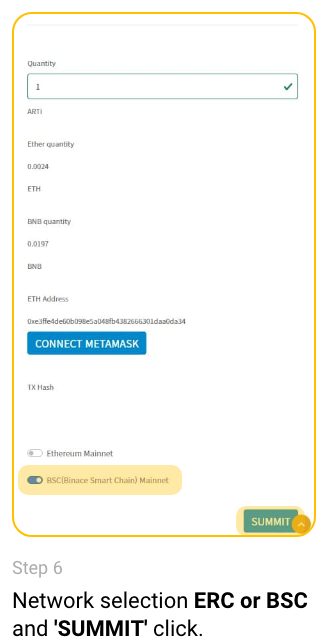
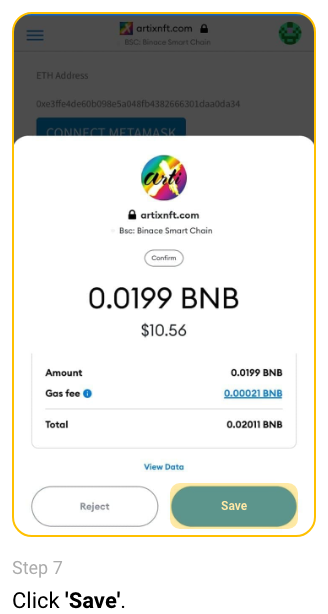
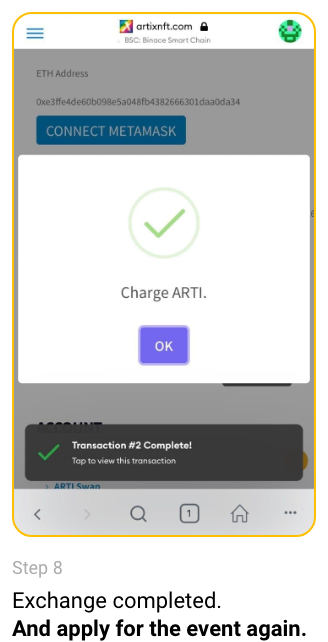
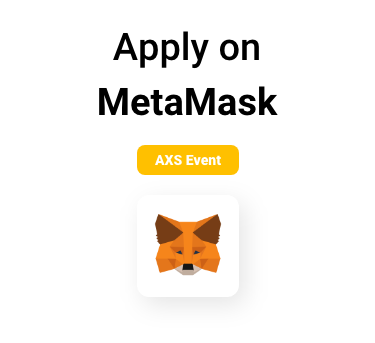
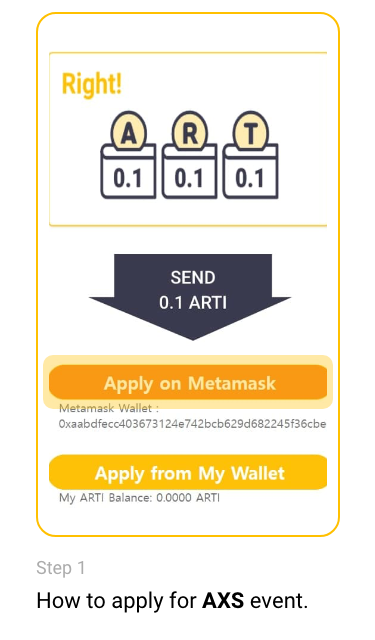
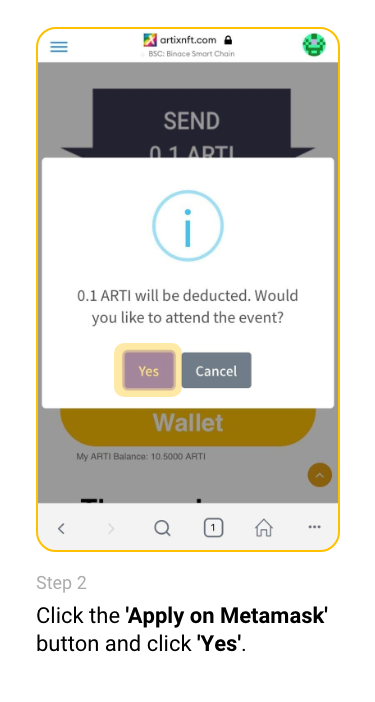
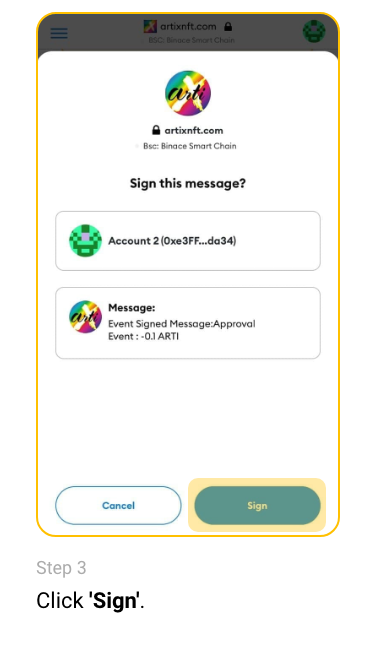
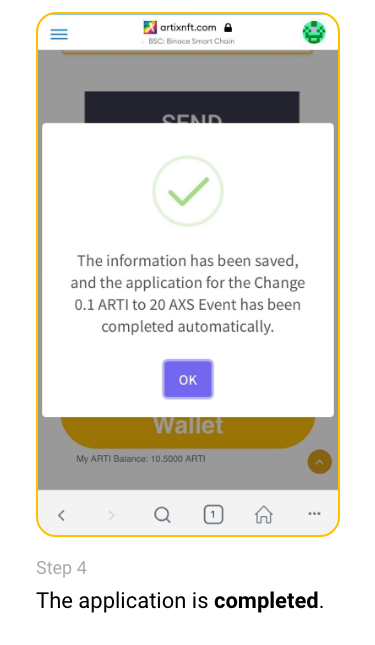
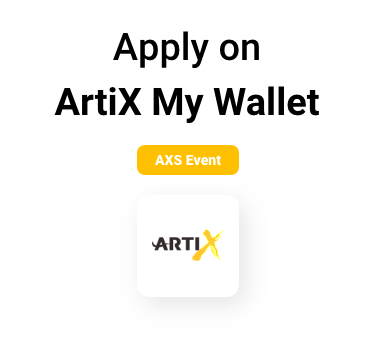
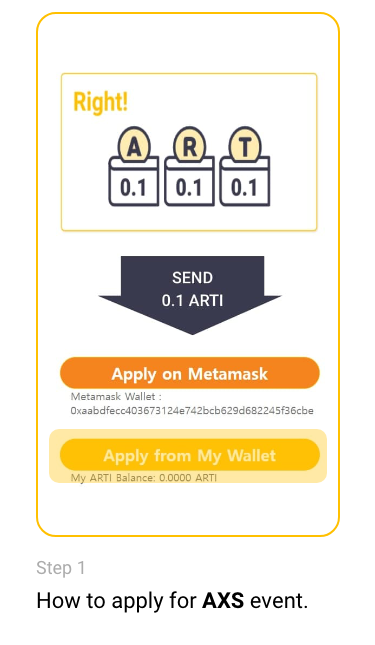
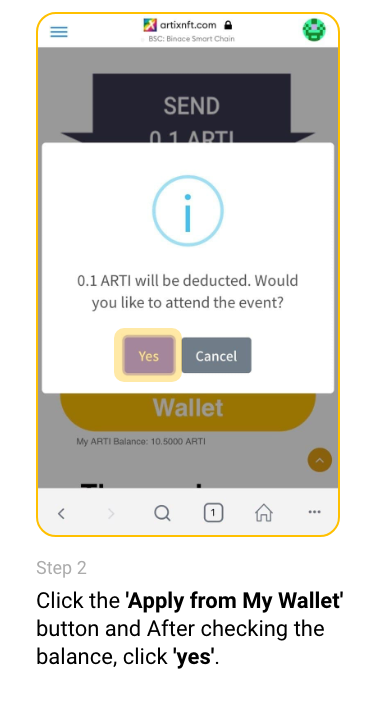
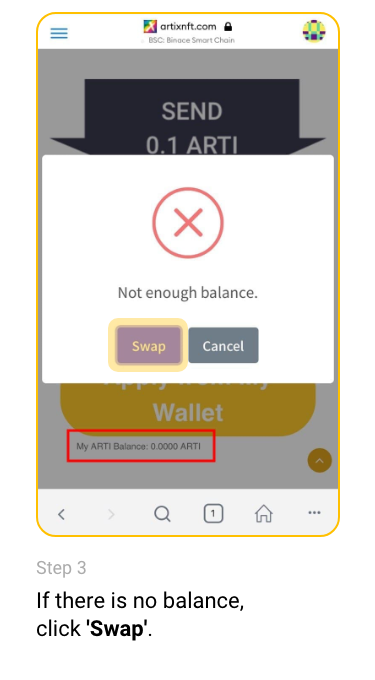
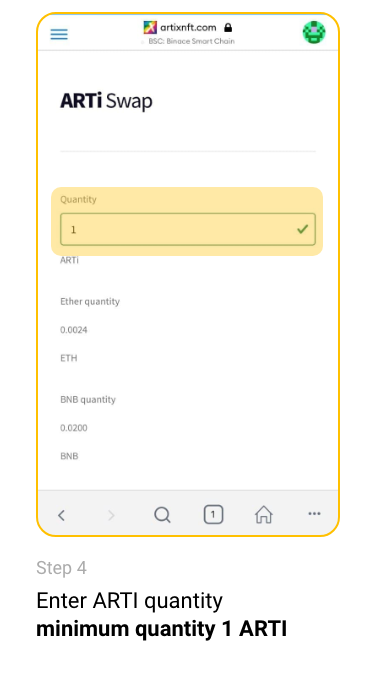
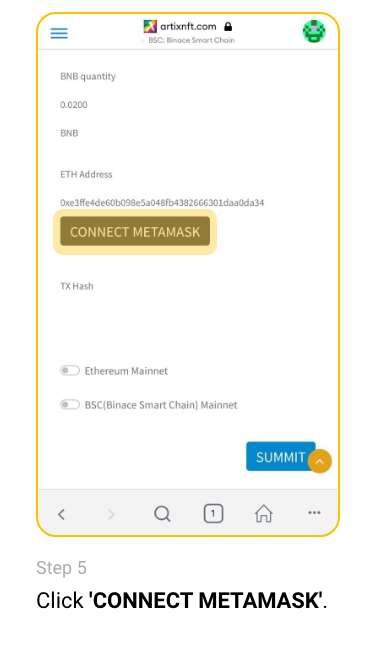
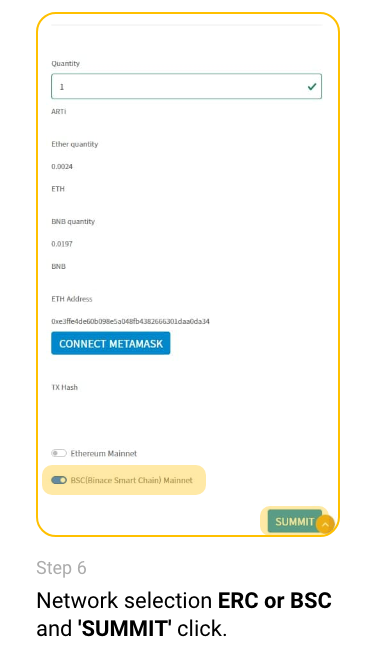
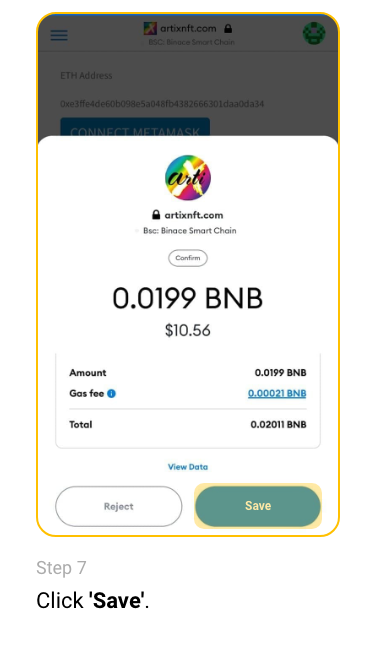
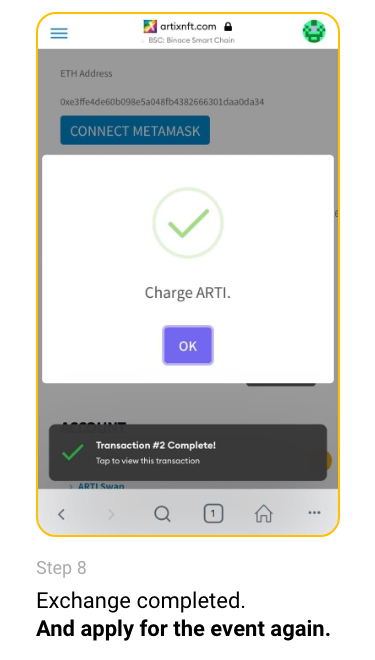
Those who applied
Those who applied